Swagger
NO!
Swagger就够了
Swagger
前端要接口文档?
NO!
直接甩给他http://xxx:8080/swagger-ui.html
~
Swagger
- 号称世界上最流行的API框架
- RestFul Api 文档在线自动生成工具 ,APi与Api定义同步更新
- 直接运行,可以在线测试API接口
- 支持多种语言:Java、PHP…
使用Swagger
- 导入依赖:
1 | <!-- spring swagger2整合 --> |
- 设置配置:
需要配置Swagger的bean实例——Docket
1 |
|
访问http://localhost:8080/swagger-ui.html
,看到我们配置的结果
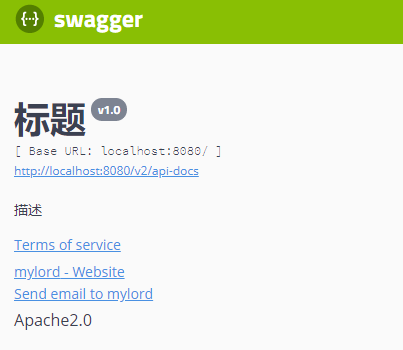
- 设置为只有
dev
环境才开启swagger
1 |
|
- 在测试中加上
TOKEN
,在docket中加上
1 | ParameterBuilder tokenParam = new ParameterBuilder(); |
相关注解
使用注解
@Api
- 功能:描述
controller
类 - 注解位置:类
- 常用注解属性
tags = "" // 描述此controller类
- 功能:描述
@ApiOperation
- 功能:描述一个方法或者一个API接口
- 注解位置:方法
- 常用注解属性
value = "" // 描述方法
notes = "" // 描述方法详细信息
@ApiImplicitParam
- 功能:描述方法或接口参数
- 注解位置:方法
- 注解属性
name = "" // 方法或接口的形参, 注意要与方法的参数名称相同
value = "" // 对参数的描述
paramType = "" // 参数传递方式,此属性的可选值 ["header", "query", "path", "body", "form"]
- header,使用
@RequestHeader
获取的参数 - query,使用
@RequestParam
获取的参数,常用于GET
请求 - path,使用
@PathVariable
获取的参数 - body,使用
@RequestBody
获取的参数,常用于POST
请求,对象参数
- header,使用
dataType = "" // 参数类型,例如 string, int, ArrayList, POJO类
@ApiImplicitParams
- 功能:汇集多个参数
- 注解位置: 方法
- 注解属性
@ApiImplicitParam
组成的列表
@ApiModel
- 功能:描述Form表单类
- 注解位置:form表单类上
@ApiModelProperty
- 功能:描述表单类的各个字段
- 注解位置:form表单类上的各个属性
- Controller
1 |
|
- Form
1 |
|
打开swagger-ui可以看到